Today, we’ll walk you through how to create constraints programmatically by building a simple mobile application’s UI completely in code without the use of Storyboards or NIBs. We will not go into the debate of which is better because, simply, they all have their pros and cons.
In part two of this PureLayout tutorial, we create some of the most used mobile app UI elements in code, including a nav bar, table view, and dynamically-sized cells.
This tutorial was written using Xcode 9 and Swift 4. we also assume that you’re familiar with Xcode, Swift, and CocoaPods.
Without further delay, let’s start building our project: a simple Contact Card application. This tutorial aims to teach you how to create constraints programmatically and build your application’s UI in code, and as such, it will not contain any logic about the application’s functionality unless it serves this tutorial’s purpose.
Setting up the project
Start by firing up Xcode -> "Create a New Xcode Project". Select "Single View App" and press "Next".
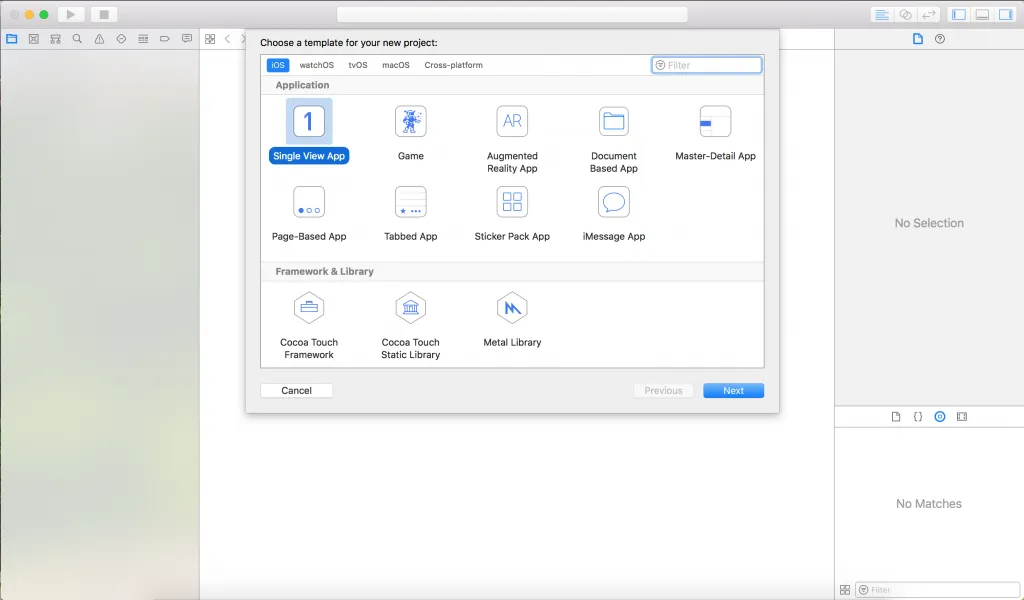
Name the project anything you’d like, I chose to call it "ContactCard", for no obvious reasons. Untick all three options below and, of course, choose Swift to be the programming language, then press "Next".
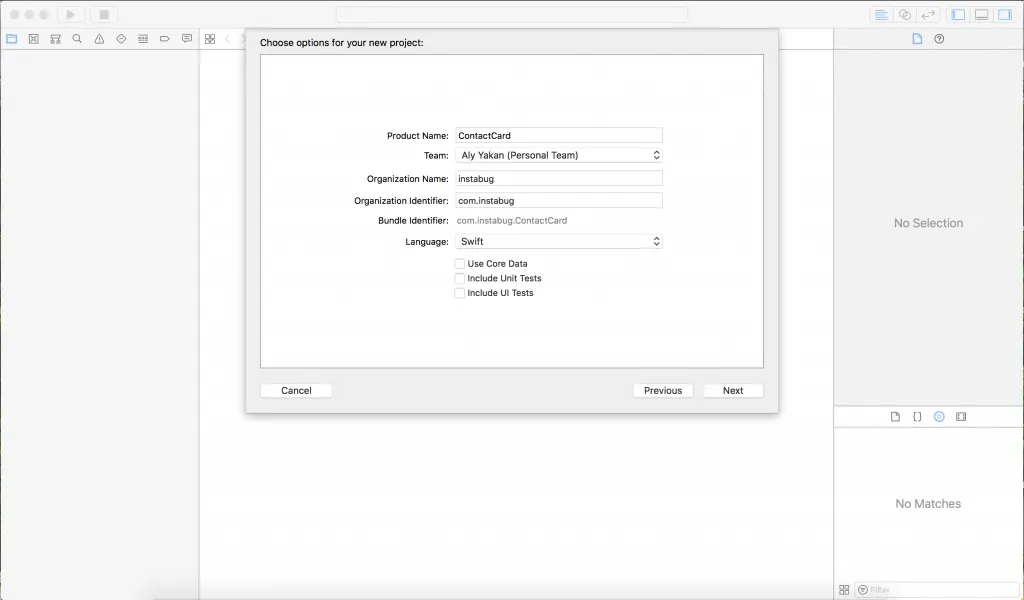
Choose a location on your computer to save the project. Uncheck "Create Git Repository on my Mac" and press "Create".
Since we won’t be using Storyboards, or NIBs for that matter, go ahead and delete "Main.storyboard", which can be found in the Project Navigator here:

After that, click on the project in the Project Navigator and under the "General" tab find the section that says "Deployment Info" and delete whatever’s written next to "Main Interface", usually it is the word "Main". This is what tells Xcode which Storyboard file to load with the application startup, but since we just deleted "Main.storyboard", leaving this line would crash the app since Xcode would not find the file.
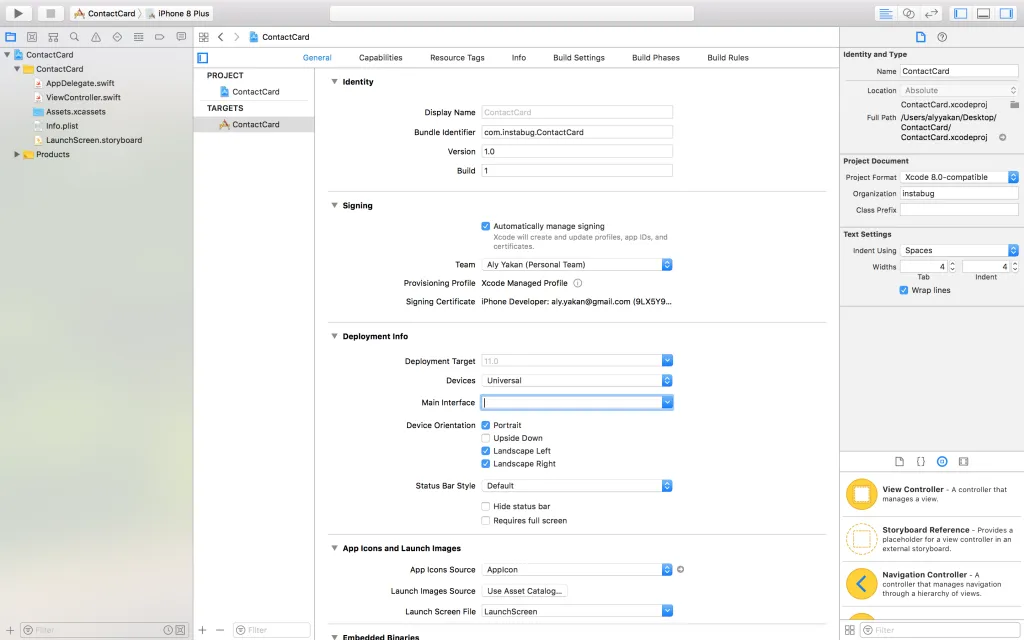
So go ahead and delete the word "Main".
Creating ViewController
Now run the application on your preferred simulator device.
A useful shortcut to navigate between files in Xcode is "⇧⌘O" and then typing the file’s name or even a piece of code that you’re looking for and a list of files will come up on the screen from which you can choose.
After running the application, this should be the result on your simulator’s screen:
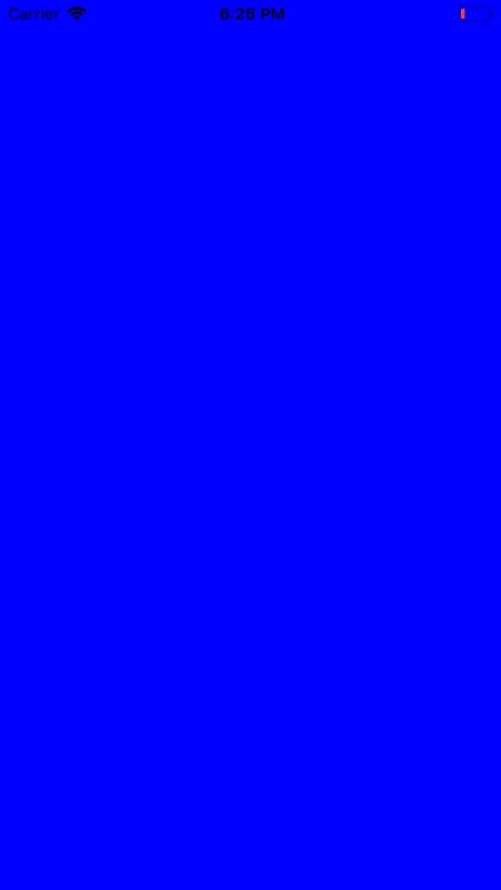
Laying out the UI
- pod init
- pod install
This should be the output of your terminal after running the second command:

pod “PureLayout”
Coffee break
Now that everything is set up, let’s start with the real work. Head over to "ViewController.swift" and grab a cup of coffee because here’s what the final result will look like:
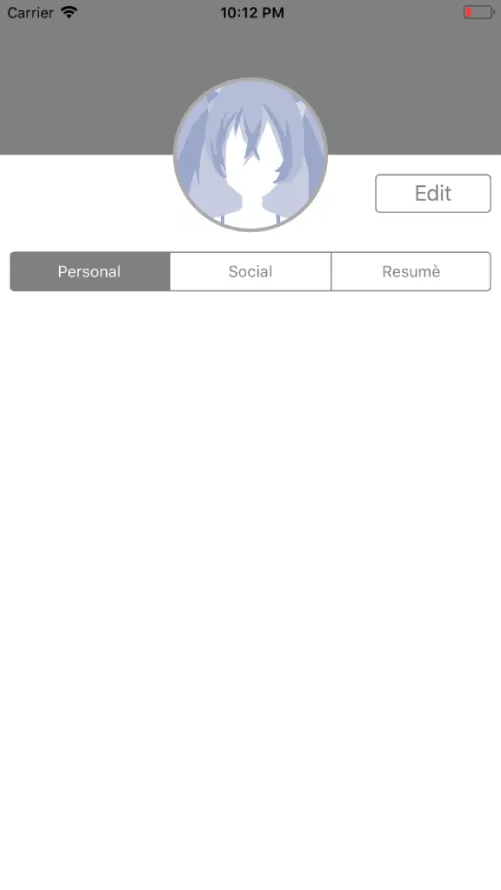
Creating an ImageView
As for the image, get any image on your desktop that you’d like to use as an avatar, draw and drop it in Xcode under <Your Project’s Name> folder, which in our case is "ContactCard", and check on "Copy items if needed".

Then click "Finish". After that, write that file’s name along with its extension inside the declaration of the UIImage instead of “avatar.jpg”.
For those of you who don’t know, lazy variables are like normal variables except they do not get initialized (or allocated any memory space) until they are needed, or being called, for the first time. This means that lazy variables don’t get initialized when the view controller is initialized, but rather they wait until a later point when they are actually needed, which saves processing power and memory space for other processes. These are especially useful in the case of initializing UI components.
PureLayout in action
Adding subviews
Setting up constraints

Bring to front
This method will bring the avatar all the way from the bottom of the stack right back to the top, no matter how many views there were above it. So choose whichever method you’d like. Of course for readability, it’s better to add the subviews in the order that they should appear above the other, if they would ever happen to intersect, while keeping in mind that the first added subview will be at the bottom of the stack and so any other intersecting view will appear on top of it.
And this is how it should really look like:

Creating segmented control
Moving on, we’ll now create the segmented control, which is the gray bar that contains three sections. Creating the segmented control is actually simple. Just do the following:

Adding a button
Now comes the last piece of UI for this small tutorial, which is the "Edit" button. Add the following lazy variable:
Now add the button as a subview like the rest of the components and add the following constraints to have it appear where it is supposed to be:
Here we only set the right and top constraints for the button, and since we gave it a size, it won’t expand and will need nothing else. Now go ahead and run the project to see the final result:
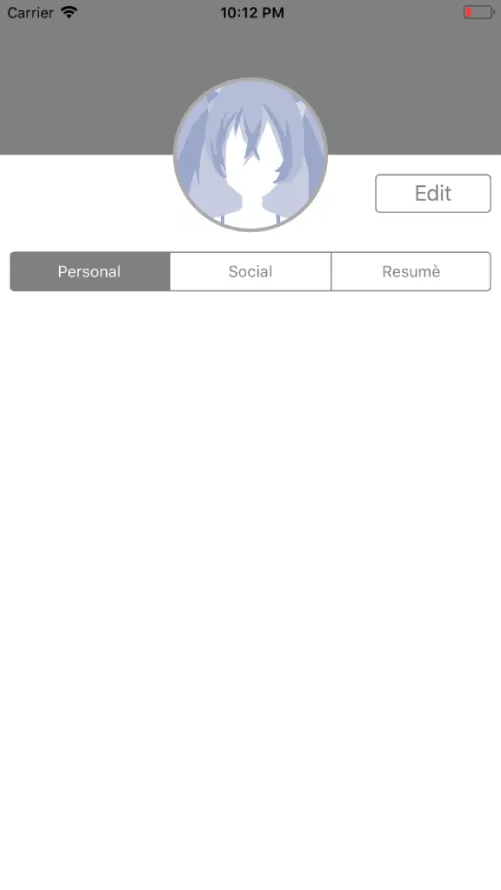
This was just a quick tutorial on how to create constraints programmatically, play around, add as many UI elements as you want. Try to re-create any application’s views that you see challenging. Start simple and build up from there. Try to draw the UI components on a piece of paper so you can imagine how they fit together.
In part two, we extend this tutorial to create a navigation bar, table view, and dynamically-sized cells in code.
Learn more:
- Creating UI Elements Programmatically Using PureLayout
- Catching Unsatisfiable Auto Layout Constraints in UITests on CircleCI
- How We Automate Our iOS Workflow at Instabug Using CircleCI
- How We Migrated Our Frontend While Scaling at Instabug
Instabug empowers mobile teams to maintain industry-leading apps with mobile-focused, user-centric stability and performance monitoring.
Visit our sandbox or book a demo to see how Instabug can help your app